1) Export Spreadsheet menu is not visible in Forms Based application in SharePoint:
Solution: Go tO -
Central Administration > Application Management >
Authentication Providers > Edit Authentication
Checked "Yes" in "Enable Client Integration? "
Notes: Client Integration
Disabling client integration will remove features which launch client applications. Some authentication mechanisms (such as Forms) don't work well with client applications. In this configuration, users will have to work on documents locally and upload their changes.
2) Apply validation controls on SharePoint's DateTimeControl
Following code snippet displays, how to apply following validations on Sharepoint's DateTimeControl:
a) Required field validation
b) Valid date validation
c) Date compare validation
<table cellpadding="0" cellspacing="0" style="font-size: 8pt; font-family: Tahoma;">
<tr>
<td>
<SharePoint:DateTimeControl ID="dateTimeStartDate" runat="server"
DateOnly="true"></SharePoint:DateTimeControl>
</td>
<td></td>
</tr>
<tr>
<td>
<SharePoint:DateTimeControl ID="dateTimeEndDate" runat="server"
DateOnly="true"></SharePoint:DateTimeControl>
</td>
<td>
<asp:RequiredFieldValidator ID="RequiredFieldValidator5" runat="server"
ControlToValidate="dateTimeEndDate$dateTimeEndDateDate"
ErrorMessage="* Required value"
Display="Dynamic"></asp:RequiredFieldValidator>
<asp:CompareValidator ID="CompareValidator2" runat="server"
ForeColor="Red"
ControlToValidate="dateTimeEndDate$dateTimeEndDateDate" Type="Date"
Operator="DataTypeCheck" ErrorMessage="* Please enter an valid date."
Display="Dynamic"></asp:CompareValidator>
<asp:CompareValidator ID="valDate" runat="server" ForeColor="Red"
ControlToValidate="dateTimeEndDate$dateTimeEndDateDate"
ControlToCompare="dateTimeStartDate$dateTimeStartDateDate" Type="Date"
Operator="GreaterThanEqual"
ErrorMessage="* Please enter End Date Greater or Equal to Start Date."
Display="Dynamic"></asp:CompareValidator>
</td>
</tr>
</table>
3) Access denied issue for Forms based user
I did following thing to solve "Access Denied" issue in "Forms Based configuration" ---
Go to :
1) Central Administration > Application Management > Policy for Web Application
2) Select proper Web Application (from top-right)
3) Click "Add User" (top-left)
4) Add required User or Role in it.
5) Grant it "Full Control" (as per requirement).
Available options:
[
Full Control - Has full control.
Full Read - Has full read-only access.
Deny Write - Has no write access.
Deny All - Has no access.
]
Then its work fine for me !!!
4) Operation with SPCoulmn Type - SPFieldChoice
Following code snippet retrieves the values stored in a SPColumn.
You can used these values to populated either Dropdown or Listbox as per you requirement.
SPList list = oWebsite.Lists["SPList_Name"];
SPFieldChoice choice = (SPFieldChoice)list.Fields["Event Name"];
foreach(string s in choice.Choices)
Response.Write(s);
5) Open SharePoint page in edit-mode
There are basically two ways to open a sharepoint page in edit-mode
a) Go to Site Actions (Top-Right) and then selecte Edit page.
b) Append the query string of current page with
?toolpaneview=2e.g. http://myserver.default.aspx?toolpaneview=2
6) Access AppSettings in SharePoint
//Web config entry
<appSettings>
<add key="UpdateTime" value="300" />
</appSettings>
//C# code to access appsetting
System.Configuration.ConfigurationSettings.AppSettings["UpdateTime"].ToString();
//or new way
System.Configuration.ConfigurationManager.AppSettings["UpdateTime"].ToString();
7) RichTextbox or InputFormTextBox in SharePoint
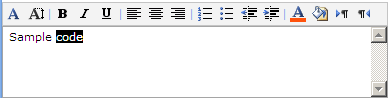
//C# code
InputFormTextBox body = new InputFormTextBox();
body.RichText = true;
body.RichTextMode = Microsoft.SharePoint.SPRichTextMode.Compatible;
body.Rows = 5;
body.TextMode = TextBoxMode.MultiLine;
body.Width = new Unit(300);
this.Controls.Add(body);
Reference--
sharepoint-using-the-rich-text-box-in-a-custom-user-control8) Session usage in SharePoint
To enable session in your SharePoint pages, open you web application web.config file.
a) Search HttpModule in cofig file, uncomment the following code -
<add name="Session" type="System.Web.SessionState.SessionStateModule" />
b) If session still doesn't work, then set EnableSessionState property to true in page directive.
After enabling the session, if you face some error like...
unable to serialize the session state. in 'stateserver' and 'sqlserver' mode asp.netThen again open you web.config file and delete following line...
<sessionState mode="StateServer" timeout="60" allowCustomSqlDatabase="true"
partitionResolverType=
"Microsoft.Office.Server.Administration.SqlSessionStateResolver,
Microsoft.Office.Server, Version=12.0.0.0, Culture=neutral,
PublicKeyToken=71e9bce111e9429c" />
9) User Profile in SharePoint
SPSite mySite = SPControl.GetContextSite(Context);
SPWeb myWeb = SPControl.GetContextWeb(Context);
ServerContext context = ServerContext.GetContext(mySite);
UserProfileManager myProfileManager = new UserProfileManager(context);
string CurrentUser = SPContext.Current.Web.CurrentUser.LoginName;
UserProfile myProfile = myProfileManager.GetUserProfile(CurrentUser);
if (myProfile[PropertyConstants.WorkEmail].Value != null)
{
oLabelWorkEmail.Text = myProfile[PropertyConstants.WorkEmail].Value.ToString();
}
References:
How to retrieve current user profile propertiesMSDN Forum10) Run the code with Elevated Privileges
While excuting the code if we face some
Privilege or
Access issue, then to solve this we can either use
SPSecurity.RunWithElevatedPrivileges OR
UserToken.
Check following code snippets...
try
{
// Get the Site ID before entering RunWithElevated...
Guid siteID = SPContext.Current.Site.ID;
SPSecurity.RunWithElevatedPrivileges(delegate()
{
using (SPSite site = new SPSite(siteID))
{
// Your code logic here,
//without calling SPContext anywhere inside this method...
}
});
}
catch (SPException spex)
{
//Log exception
}
Note - It is very important to not call the SPContext from within any elevated method, as it'll result in your elevated privileges to cease.
SPUserToken sysToken = SPContext.Current.Site.SystemAccount.UserToken;
using (var spSite = new SPSite(SPContext.Current.Site.ID, sysToken))
{
using (var spWeb = spSite.OpenWeb(SPContext.Current.Web.ID))
{
//Your code
}
}